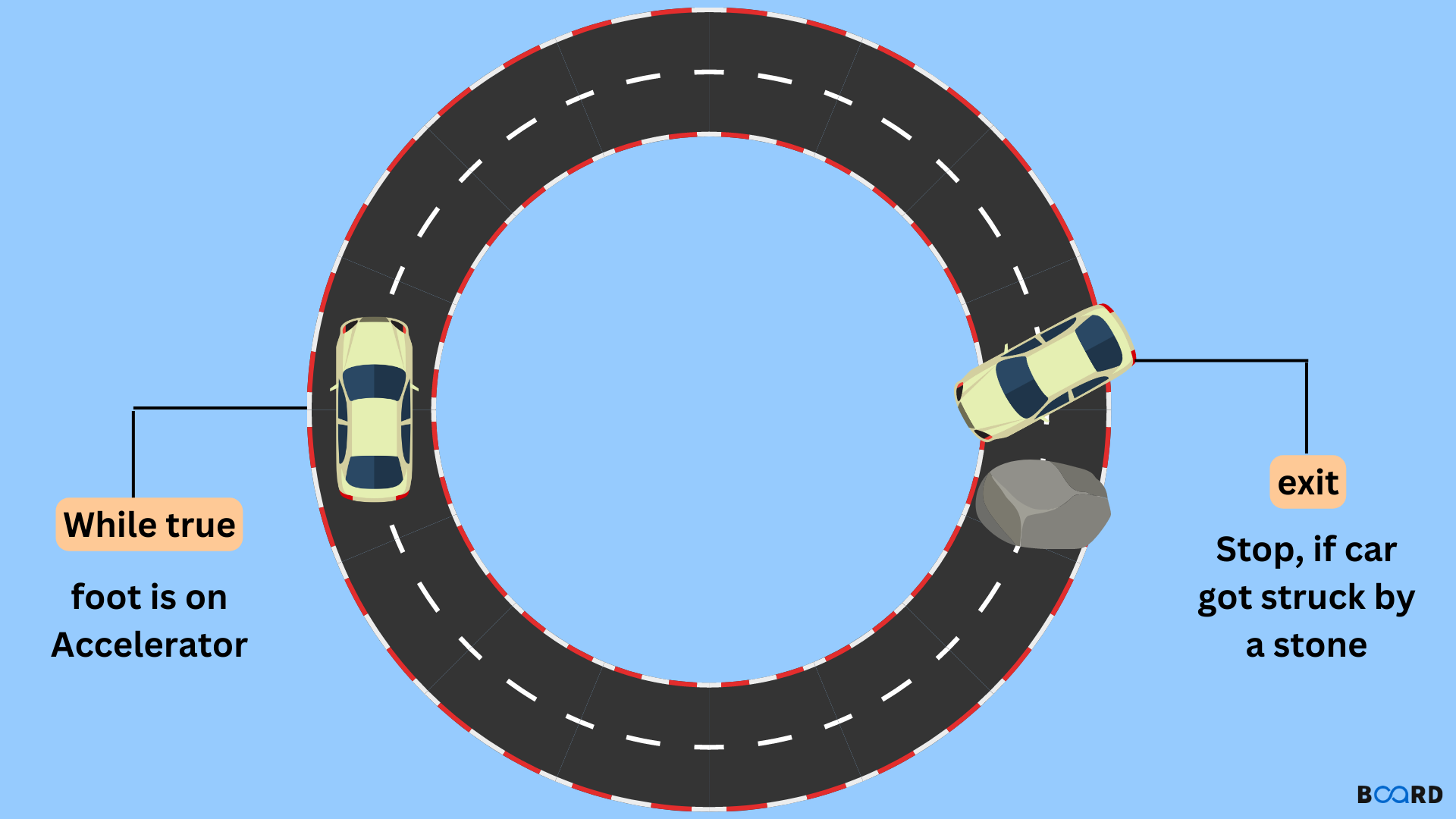
Introduction
"While True" is a looping construct in the Python programming language that allows a block of code to be repeated indefinitely. It is often used in conjunction with a break statement, which allows the loop to be exited under certain conditions.
To use a "while True" loop in Python, you must first define a condition that will eventually evaluate to "False" for the loop to terminate. This is typically done using a Boolean variable initially set to "True," then modified within the loop to become "False".
Example 1
Here is an example of a basic "while True" loop in Python:
As written, this loop will print the message "This loop will run forever!" indefinitely. To exit the loop, we can use a break statement, which will cause the loop to terminate when a certain condition is met.
Example 2
Here is an example of a "while True" loop that uses a break statement to exit the loop when a certain condition is met:
In this example, the loop will print the message "This loop will run forever!" ten times before exiting. This is because the counter variable is incremented by 1 each time the loop runs, and the break statement is executed when the counter reaches 10.
It is important to note that "while True" loops should be used cautiously, as they can run indefinitely if the break statement is not properly implemented. This can cause performance issues and even crash the program if the loop consumes too many resources.
One common use for "while True" loops are creating infinite scroll pages, where new content is loaded as the user scrolls down the page. In this case, the loop is used to check for new content continuously, and the break statement is used to exit the loop when the user reaches the end of the available content.
Example 3
Here is an example of a "while True" loop that is used to load new content as the user continuously scrolls down a page:
In this example, the loop will run indefinitely, calling the "get_new_content()" function each time to check for new content. If new content is available, it is appended to the page using the "append_to_page()" function. If no new content is available, the break statement is executed, and the loop is terminated.
Another common use for "while True" loops are creating command-line tools and interactive programs. In these cases, the loop continuously accepts and processes user input until the program is terminated.
Here is an example of a "while True" loop that is used to accept and process user input in a command-line program continuously:
In this example, the loop will run indefinitely, accepting and processing user input each time it runs. If the user enters the "exit" command, the break statement is executed, and the loop is terminated. A list of available commands is displayed iff the user enters the "help" command. If the user enters the "list" command, a list of items is displayed using the "display_items()" function. If the user enters the "add" command, the user is prompted to enter a new item, which is then added to the list using the "add_item()" function. If the user enters any other command, an error message indicates that the command is invalid.
Conclusion
In conclusion, the "while True" loop is a useful tool in Python programming that allows a block of code to be repeated indefinitely. It is often used with a break statement to exit the loop under certain conditions. "While True" loops should be used cautiously, as they can run indefinitely if the break statement is not properly implemented. They are commonly used to create infinite scroll pages and interactive programs, where they are used to continuously check for new content or accept and process user input. You can create more dynamic and interactive programs in Python by understanding how to use "while True" loops effectively.